A Beginner’s Guide to Python for Data Science - Part 4 Statements and Indentation in Python
In the last article, we introduced the jupyter notebook and its functionality.
Statements:
A Statement is any instruction or set of commands given to Python, or we can say that a statement is any code executable inside Python.
So a simple addition that we performed while checking the installation is an example of a statement.
There are two types of statements that you would generally see in Python, they are:
- Single-line statement.
- Multi-line statement.
Let’s see an example of a multi-line statement. We will add the first six numbers for the task.
We will run the following command:
my_variable = (1 + 2 + 3 + 4 + 5 + 6)
As soon as we print the variable we see that we get the sum of desired numbers.
In the above code, I entered the entire addition in one line, which could also have been split into multi-line, as shown below:
my_variable = ( 1 + 2
+ 3 + 4
+ 5 + 6)
If we run the above code, we will not receive any error from Python because the brackets mentioned in the code suggest to Python that the code is not complete yet.
If we run the same code without brackets, then you will find Python throwing a syntax error, as shown below:

my_variable = 1 + 2 \
+ 3 + 4 \
+ 5 + 6
Here the backslash works as the symbol, which informs Python that the code line is not complete yet and is an example of a multi-line statement.
Indentation:
If you have some prior experience coding in other languages, you would know that indentation is required to make our codes more readable. It is a requirement when we want to make our code look neat and clean.
In Python, indentation has a different use. It is a way by which we inform Python about differentiating between the various code blocks.
Think of it like this; if you have coded in C or C++, you would be aware of the curly braces that differentiate between the different code blocks. We use the white spaces for the same task as the curly braces inside Python. So we should be careful while putting any random indentation/space inside the code as it could break the flow of the code.
Generally, we use white spaces or tabs for indentation based on personal preferences. Some people use white space using the space bar, whereas others use the tab key for initiating the indentation in Python.
Let’s see a small example to see how we apply indentation.
Suppose we want to print the first ten numbers using a for loop in Python.
We will run the following code to achieve this:
for i in range(1, 11):
print(i)
In the above code, we used an inbuilt function called “range”, which gives us values from 1 to 10. We are using a for loop to print the numbers one by one, as shown below:
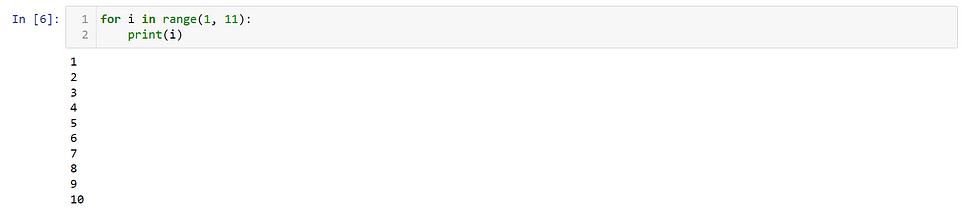
Here, you can see that when you write the colon sign after the range function and press enter, Python automatically gives an indentation when we start writing the print statement, as shown in the above image.
Let’s see what happens if someone doesn’t follow the indentation rule. We will use the above example for printing the ten numbers. This time we remove the indentation which gets created and run the code, as shown below:

#Python #Data Science #Data Science Training #Learn Python
Comments
Post a Comment